In this blog, you are going to learn how to paginate database results in CodeIgniter using the pagination library.
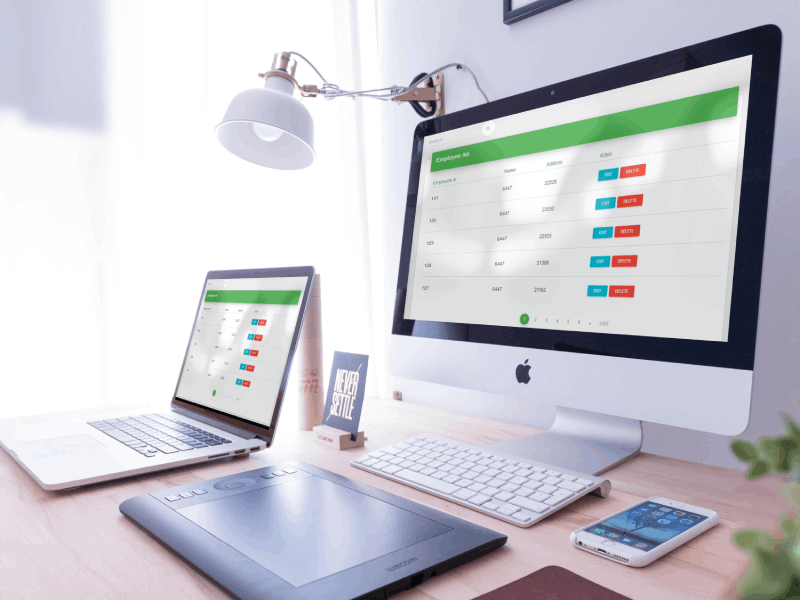
Step 1: Create one table like `EMPLOYEE_DATA`
CREATE TABLE `EMPLOYEE_MASTER_DATA` (
`ID` int(11) NOT NULL AUTO_INCREMENT,
`EMP_NO` varchar(50) DEFAULT NULL,
`EMP_NAME` varchar(255) DEFAULT NULL,
`ADDRESS` text DEFAULT NULL,
PRIMARY KEY (`ID`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Step 2: Insert some records in `EMPLOYEE_DATA` table
--
-- Dumping data for table `EMPLOYEE_DATA`
--
INSERT INTO `EMPLOYEE_DATA` (`ID`, `EMP_NO`, `EMP_NAME`, `ADDRESS`) VALUES
(1, '101', 'Divyesh Patel', 'Valsad, Gujarat'),
(2, '102', 'Roshni Patel', 'Chikhli, Gujarat'),
(3, '103', 'Urvish Patel', 'Gandevi, Gujarat'),
(4, '101', 'Niral Patel', 'Valsad, Gujarat'),
(5, '1101', 'Divyesh Patel', 'Valsad, Gujarat'),
(6, '1102', 'Roshni Patel', 'Chikhli, Gujarat'),
(7, '1103', 'Urvish Patel', 'Gandevi, Gujarat'),
(8, '101', 'Shinerweb', 'Valsad, Gujarat'),
(9, '102', 'Google', 'Chikhli, Gujarat'),
(10, '103', 'Facebook', 'Gandevi, Gujarat'),
(11, '101', 'Shinerweb', 'Valsad, Gujarat'),
(12, '102', 'Google', 'Chikhli, Gujarat'),
(13, '103', 'Facebook', 'Gandevi, Gujarat'),
(14, '101', 'Divyesh Patel', 'Valsad, Gujarat'),
(15, '102', 'Roshni Patel', 'Chikhli, Gujarat'),
(16, '103', 'Urvish Patel', 'Gandevi, Gujarat');
Step 3: Create one model file like pagination_model.php
to get data from the database table
In this file we create two function allrecord()
and data_list()
. First function is use for count number of records in the table and second function is use for fetch offset wise data and limited data per page.
<?php
class pagination_model extends CI_Model
{
//--- Get total record
public function allrecord($title){
if(!empty($title)){
$this->db->like('EMP_NAME',$title);
}
$this->db->select('ID');
$this->db->from('EMPLOYEE_DATA');
//$this->db->where('DELETE_STATUS','N'); //IF YOU WANT WHERE CONDITION THEN USE THIS CODE
$rs = $this->db->get();
$rs->num_rows();
return $rs->num_rows();
}
//--- fetch record list with search offset
public function data_list($limit,$offset,$title){
if(!empty($title)){
$this->db->like('EMP_NAME',$title);
}
$this->db->select('ID,EMP_NO,EMP_NAME,ADDRESS');
$this->db->from('EMPLOYEE_DATA');
//$this->db->where('DELETE_STATUS','N'); //IF YOU WANT WHERE CONDITION THEN USE THIS CODE
$this->db->order_by('ID','DESC');
$this->db->limit($limit,$offset);
$rs = $this->db->get();
return $rs->result();
}
}
?>
Step 4: Create one controller file like pagination.php
In this controller file first you need to load library $this->load->library("pagination");
in constructor and create one function index()
and write below logic for pagination.
<?php
class pagination extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->helper(array('form', 'url'));
$this->load->library("pagination");
$this->load->model('pagination_model');
}
// ---- display register list
function index()
{
if($this->input->post('title') !="")
{
$title = trim($this->input->post('title'));
}
else{
$title = str_replace("%20",' ',($this->uri->segment(3))?$this->uri->segment(3):0);
}
$data['search_title']=$title;
$allrecord = $this->pagination_model->allrecord($title);
$baseurl = base_url().'index.php/'.$this->router->class.'/'.$this->router->method."/".$title;
$paging=array();
$paging['base_url'] =$baseurl;
$paging['total_rows'] = $allrecord;
$paging['per_page'] = 5;
$paging['uri_segment']= 4;
$paging['num_links'] = 5;
//config for bootstrap pagination class integration
$paging['full_tag_open'] = '<ul class="pagination pagination-success">';
$paging['full_tag_close'] = '</ul>';
$paging['first_link'] = 'First';
$paging['last_link'] = 'Last';
$paging['first_tag_open'] = '<li>';
$paging['first_tag_close'] = '</li>';
$paging['prev_link'] = '«';
$paging['prev_tag_open'] = '<li class="prev">';
$paging['prev_tag_close'] = '</li>';
$paging['next_link'] = '»';
$paging['next_tag_open'] = '<li>';
$paging['next_tag_close'] = '</li>';
$paging['last_tag_open'] = '<li>';
$paging['last_tag_close'] = '</li>';
$paging['cur_tag_open'] = '<li class="active"><a href="#">';
$paging['cur_tag_close'] = '</a></li>';
$paging['num_tag_open'] = '<li>';
$paging['num_tag_close'] = '</li>';
$this->pagination->initialize($paging);
$data['limit'] = $paging['per_page'];
$data['number_page'] = $paging['per_page'];
$data['offset'] = ($this->uri->segment(4)) ? $this->uri->segment(4):'0';
$data['links'] = $this->pagination->create_links();
$data['data_list'] = $this->pagination_model->data_list($data['limit'],$data['offset'],$title);
$this->load->view('pagination_list_view',$data);
}
}
?>
Step 5: Create views file like pagination_list_view.php
to load record with pagination
In a view file will display recent five records by using foreach()
loop and display pagination with this code <?php echo $links; ?>
. We also added search code with pagination in below code.
<div class="row">
<div class="col-lg-6 col-md-6 col-sm-8">
<?php echo form_open(base_url().'index.php/pagination'); ?>
<table class="search_bar">
<tr>
<td>
<div class="form-group is-empty" style="margin:0px !important;">
<input type="text" id="title" name="title" value="<?php if(!empty($search_title)){ echo $search_title; } ?>" class="form-control" placeholder="Search" >
<span class="material-input"></span>
</div>
</td>
<td>
<button type="submit" class="btn btn-white btn-round btn-just-icon d-inline-block">
<i class="material-icons">search</i>
<div class="ripple-container"></div>
</td>
</tr>
</table>
<?php echo form_close(); ?>
</form>
</div>
</div>
<div class="row">
<div class="col-lg-12">
<div class="card">
<div class="card-header" data-background-color="green">
<h4 class="title">Employee list </h4>
</div>
<div class="card-content table-responsive">
<div class="row">
<div class="col-lg-12" id="responsive_table">
<table class="table table-hover" >
<thead class="text-primary">
<tr>
<th>Record no</th>
<th>Employee #</th>
<th>Name</th>
<th>Address</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<?php
if(isset($data_list) && !empty($data_list))
{
foreach($data_list as $data_list_row)
{
?>
<tr>
<td data-label="Record no"><?php echo $data_list_row->ID; ?></td>
<td data-label="Employee #"><?php echo $data_list_row->EMP_NO; ?></td>
<td data-label="Name"><?php echo $data_list_row->EMP_NAME; ?></td>
<td data-label="Address"><?php echo $data_list_row->ADDRESS; ?></td>
<td data-label="Action">
<a href="#" class="btn btn-info btn-sm" >Edit</a>
<a href="#" class="btn btn-danger btn-sm" >Delete</a>
</td>
</tr>
<?php
}
}
else
{
echo '<tr><td colspan="4" align="center">No record found.</td></tr>';
}
?>
</tbody>
</table>
<center>
<p><?php echo $links; ?></p>
</center>
</div>
</div><!-- end row -->
</div>
</div>
</div>
</div><!-- end row -->
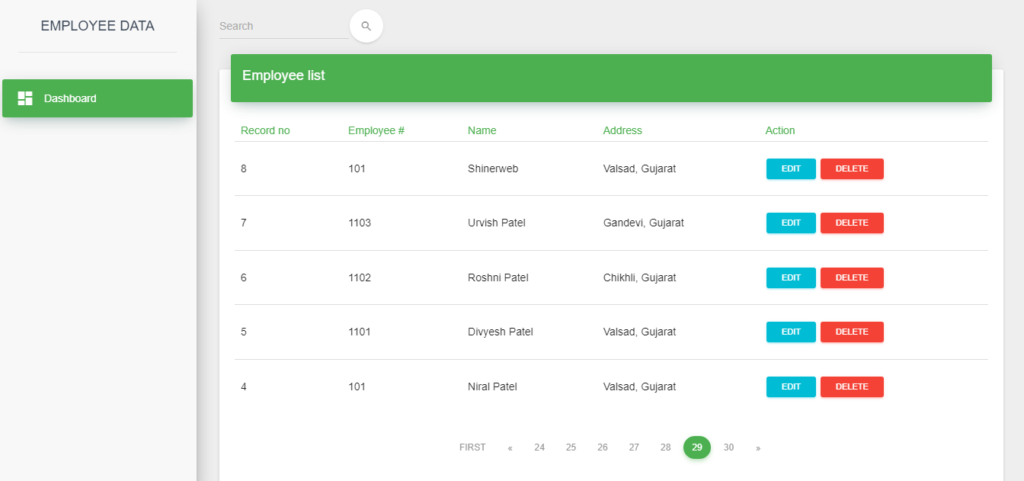