By using JsBarcode JavaScript library we will generate barcode and this is a customizable barcode generator which is helpful to create your barcode. You can also download this barcode in PNG format and you can use that barcode image wherever you want.
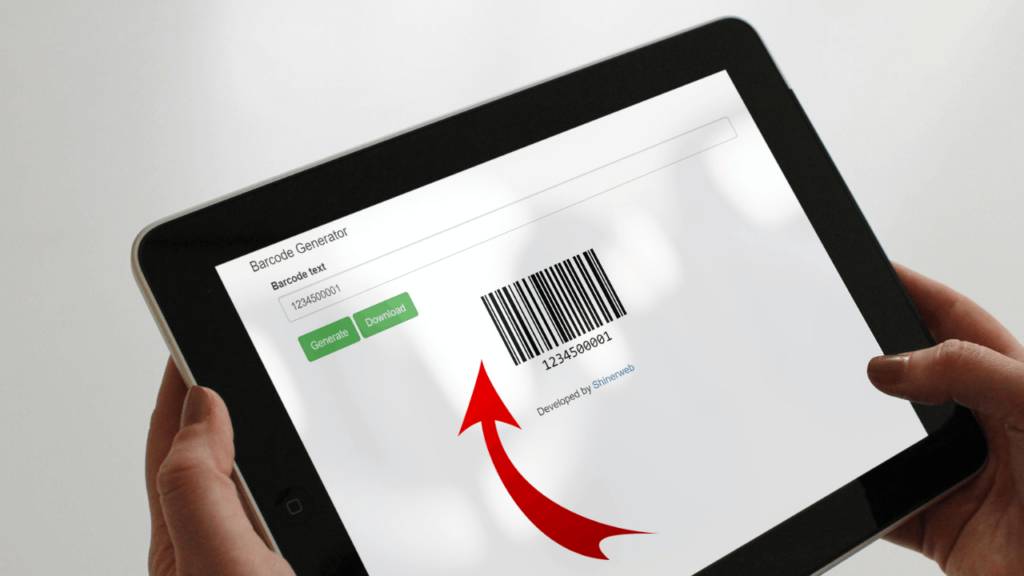
Step 1: Create a html file
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!--
Include CSS and JS library
-->
<title>Barcode Generator</title>
</head>
<body>
<!-- Barcode HTML Code -->
<script>
<!-- Barcode Generate and download Code -->
</script>
</body>
</html>
Step 2 : Include the library files in the head tag
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<!-- JsBarcode library -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/JsBarcode.all.min.js"></script>
<title>Barcode Generator</title>
</head>
In above code we have included Bootstrap library for designing your web page and here we also include jQuery to make code simple.
The most important JsBarcode JavaScript library to generate barcode for the web.
Step 3: Design your web page
<body>
<div class="container">
<h4>Barcode Generator</h4>
<div class="container">
<div class="row">
<div class="col-lg-4">
<label>Barcode text</label>
<input type="text" class="form-control" id="input" placeholder="Enter the data">
<br>
<input type="button" class="btn btn-success" onclick="generateBarcode()" value="Generate">
<input type="button" class="btn btn-success" onclick="downloadBarcode()" value="Download">
</div>
<div class="col-lg-8" align="center">
<svg style="display:none" id="barcode"></svg>
</div>
</div>
</div>
In above code we have created one textbox where we will take user input and based on that we will generate barcode. Here also we take two button one is Generate and second is Download.
When you click on Generate button we will can one JavaScript function generateBarcode() to generate barcode and that barcode will generate in SVG format. Once your barcode generated and we need to download that barcode in image format then we will call downloadBarcode() JavaScript function.
Step 4: Create a JavaScript function which will create the barcode based on the user input
<script>
function generateBarcode() {
var input = document.getElementById("input").value;
var barcode = document.getElementById("barcode");
if (input=='')
{
alert("<<!-- Provide the data first --!>> ");
return false;
}
else{
JsBarcode(barcode, input);
barcode.style.display = "inline";
}
}
</script>
In above code we will textbox value and that value pass into the JsBarcode() method and after that we will display that barcode.
Step 5: Download barcode
<script>
function downloadBarcode(e){
const canvas = document.createElement("canvas");
const svg = document.getElementById("barcode");
const base64doc = btoa(unescape(encodeURIComponent(svg.outerHTML)));
const w = parseInt(svg.getAttribute('width'));
const h = parseInt(svg.getAttribute('height'));
const img_to_download = document.createElement('img');
img_to_download.src = 'data:image/svg+xml;base64,' + base64doc;
console.log(w, h);
img_to_download.onload = function () {
console.log('img loaded');
canvas.setAttribute('width', w);
canvas.setAttribute('height', h);
const context = canvas.getContext("2d");
//context.clearRect(0, 0, w, h);
context.drawImage(img_to_download,0,0,w,h);
const dataURL = canvas.toDataURL('image/png');
if (window.navigator.msSaveBlob) {
window.navigator.msSaveBlob(canvas.msToBlob(), "download.png");
e.preventDefault();
} else {
const a = document.createElement('a');
const my_evt = new MouseEvent('click');
a.download = 'download.png';
a.href = dataURL;
a.dispatchEvent(my_evt);
}
//canvas.parentNode.removeChild(canvas);
}
}
</script>
Here we created one function to download your barcode which is generated in SVG format. When you click on Download button at that time above JavaScript code will used.