When your page have lots of content and you will scroll the page down to see all these content and if you want to go to top of the page so at that time this Scroll back to top button will be useful.
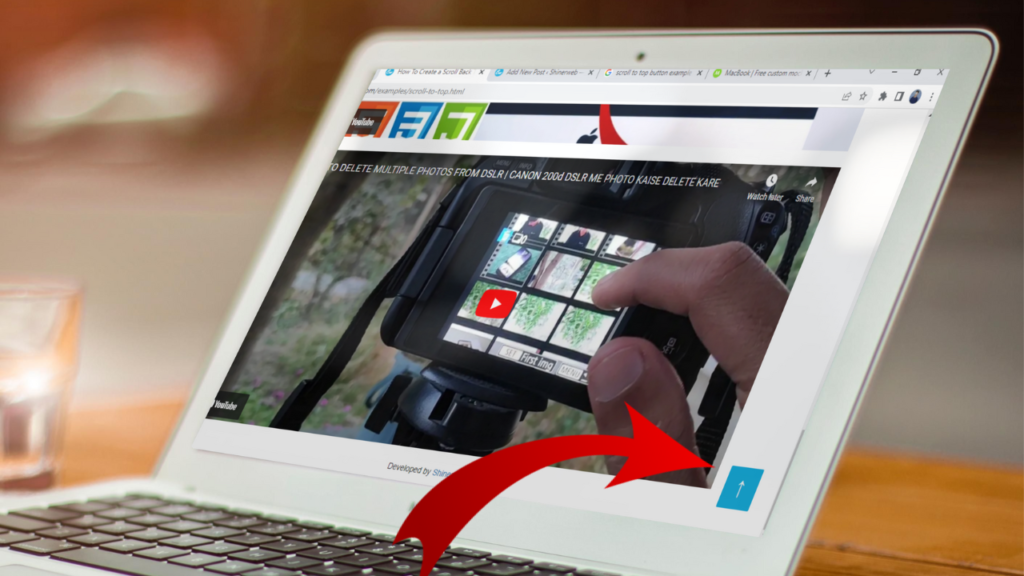
Here we will create one live demo example to create scroll back to the top of page and also will explain step by step how to configure and which CSS and Java Script required.
Step 1: Create one simple HTML file and include below library.
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Required library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<!-- Bootstrap theme -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<title>How To Create a Scroll Back To Top Button with Live Example</title>
</head>
Write above code inside <head> tag and above libraries are used to design your page with bootstrap framework.
Step 2: Add some custom CSS.
<style type="text/css">
#back-to-top.show-icon {
opacity: 1;
}
#back-to-top {
font-size: 30px;
position: fixed;
bottom: 40px;
right: 40px;
z-index: 9999;
width: 64px;
height: 64px;
text-align: center;
line-height: 60px;
background: rgb(19 174 223);
color: #fff;
cursor: pointer;
border: 0;
border-radius: 2px;
text-decoration: none;
transition: opacity 0.2s ease-out;
opacity: 0;
}
</style>
Step 3: Set up your content and put a button at the end of the body tag.
<body>
<!-- your content start -->
.
.
.
.
<!-- your content end -->
<a href="#" id="back-to-top" title="Back to top">↑</a>
</body>
Step 4: Finally write some JavaScript code.
<script>
if ($('#back-to-top').length) {
var scrollTrigger = 100, // px
backToTop = function() {
var scrollTop = $(window).scrollTop();
if (scrollTop > scrollTrigger) {
$('#back-to-top').addClass('show-icon');
} else {
$('#back-to-top').removeClass('show-icon');
}
};
backToTop();
$(window).on('scroll', function() {
backToTop();
});
$('#back-to-top').on('click', function(e) {
e.preventDefault();
$('html,body').animate({
scrollTop: 0
}, 700);
});
}
</script>
You can write above code after the body tag and this code will be run while you click on Scroll to Top Button. By using this javascript code we will show one button while you scroll down and when you click on that button it will go to the top of the page.
Here we are sharing live demo link and also you can download whole code file with below download button.