In this blog we will add a watermark on a PDF using TCPDF. Adding a watermark to a PDF document is a basic need in document editing work. TCPDF is a fantastic library for working with PDF documents in PHP.
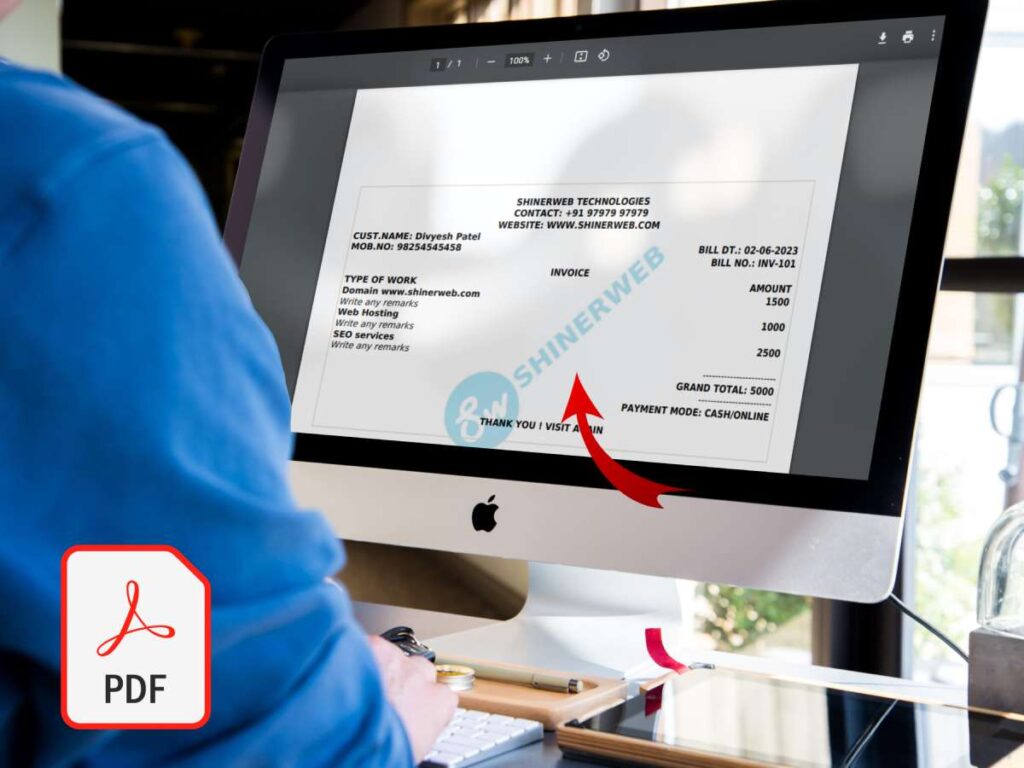
Here you can follow these steps to generate watermark on PDF:
Step 1: Create one project folder like “generate_pdf“
Step 2: Download TCPDF library
Include above downloaded library inside your project folder
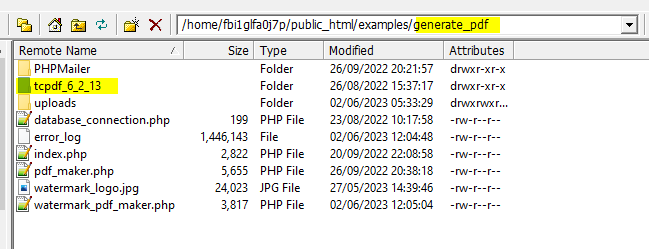
Step 3: Create one PHP file like watermark_pdf_maker.php
First we need to include TCPDF library as below
include_once('tcpdf_6_2_13/tcpdf/tcpdf.php');
Once you include the TCPDF library you required to override header() method with below code
class MyCustomPDFWithWatermark extends TCPDF {
public function Header() {
// Get the current page break margin
$bMargin = $this->getBreakMargin();
// Get current auto-page-break mode
$auto_page_break = $this->AutoPageBreak;
// Disable auto-page-break
$this->SetAutoPageBreak(false, 0);
// Define the path to the image that you want to use as watermark.
$img_file = 'https://shinerweb.com/examples/generate_pdf/watermark_logo.jpg';
//$img_file = './watermark_logo.jpg'; //when you run this code locally
// Render the image
//$this->Image( filename, left, top, width, height, type, link, align, resize, dpi, align, ismask, imgmask, border, fitbox, hidden, fitonpage);
$this->Image($img_file, 0, 10, 0, 0, '', '', '', false, 300, 'C', false, false, 0);
// Restore the auto-page-break status
$this->SetAutoPageBreak($auto_page_break, $bMargin);
// Set the starting point for the page content
$this->setPageMark();
}
}
In above code you must have to define path to the image that you want to use as watermark. Here we have used below image as a watermark.
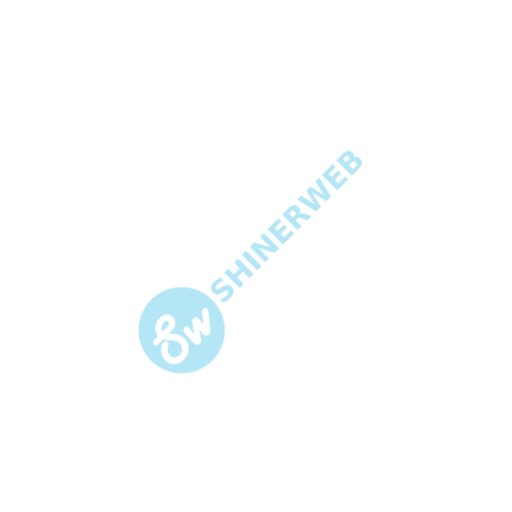
You can set all the settings of image with below parameters
//$this->Image( filename, left, top, width, height, type, link, align, resize, dpi, align, ismask, imgmask, border, fitbox, hidden, fitonpage);
Step 4: Set up the PDF document properties, such as the author, font size and orientation:
// Create a new PDF but using our own class that extends TCPDF
$pdf = new MyCustomPDFWithWatermark(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// set document information
$pdf->SetAuthor('Shinerweb');
// set default monospaced font
$pdf->SetDefaultMonospacedFont(PDF_FONT_MONOSPACED);
// set default header data
$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE.' 006', PDF_HEADER_STRING);
// set margins
$pdf->SetMargins('10', '40', '10');
$pdf->SetAutoPageBreak(TRUE, PDF_MARGIN_BOTTOM);
$pdf->SetFont('dejavusans', '', 10);
// Add a page
$pdf->AddPage();
Step 5: Write HTML code for PDF
$content = '';
$content .= '
<style type="text/css">
body{
font-size:12px;
line-height:24px;
font-family:"Helvetica Neue", "Helvetica", Helvetica, Arial, sans-serif;
color:#000;
}
</style>
<table cellpadding="0" cellspacing="0" style="border:1px solid #ddc;width:100%;">
<table style="width:100%;" >
<tr><td colspan="2"> </td></tr>
<tr><td colspan="2" align="center"><b>SHINERWEB TECHNOLOGIES</b></td></tr>
<tr><td colspan="2" align="center"><b>CONTACT: +91 97979 97979</b></td></tr>
<tr><td colspan="2" align="center"><b>WEBSITE: WWW.SHINERWEB.COM</b></td></tr>
<tr><td colspan="2"><b>CUST.NAME: Divyesh Patel </b></td></tr>
<tr><td><b>MOB.NO: 98254545458 </b></td><td align="right"><b>BILL DT.: '.date("d-m-Y").'</b> </td></tr>
<tr><td> </td><td align="right"><b>BILL NO.: INV-101</b></td></tr>
<tr><td colspan="2" align="center"><b>INVOICE</b></td></tr>
<tr class="heading" style="background:#eee;border-bottom:1px solid #ddd;font-weight:bold;">
<td>
TYPE OF WORK
</td>
<td align="right">
AMOUNT
</td>
</tr>
<tr class="itemrows">
<td><b>Domain www.shinerweb.com</b><br><i>Write any remarks</i></td>
<td align="right"><b>1500</b></td>
</tr>
<tr class="itemrows">
<td><b>Web Hosting</b><br><i>Write any remarks</i></td>
<td align="right"><b>1000</b></td>
</tr>
<tr class="itemrows">
<td><b>SEO services</b><br><i>Write any remarks</i></td>
<td align="right"><b>2500</b></td>
</tr>
<tr class="total"><td colspan="2" align="right">------------------------</td></tr>
<tr><td colspan="2" align="right"><b>GRAND TOTAL: 5000</b></td></tr>
<tr><td colspan="2" align="right">------------------------</td></tr>
<tr><td colspan="2" align="right"><b>PAYMENT MODE: CASH/ONLINE </b></td></tr>
<tr><td colspan="2"> </td></tr>
<tr><td colspan="2" align="center"><b>THANK YOU ! VISIT AGAIN</b></td></tr>
<tr><td colspan="2"> </td></tr>
</table>
</table>';
Here we design invoice copy with HTML code and that all the code stored in $content variable.
Step 6: Generate PDF
$pdf->writeHTML($content);
$file_name = "INV_001.pdf";
ob_end_clean();
$pdf->Output($file_name, 'I'); // I means Inline view
//$pdf->Output($file_name, 'D'); // D means download
In above code we have used writeHTML() and Output() method to generate PDF.