In this blog we will create on live demo example where we can implement live autocomplete search by using autocomplete widget of jQuery UI and PHP.
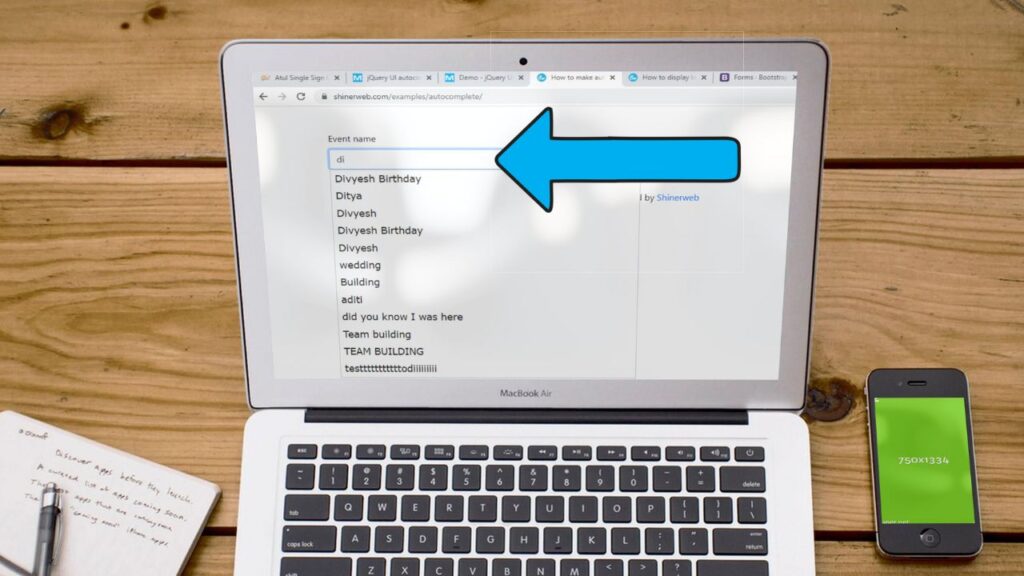
In this tutorial, I will explain all the process and setups for autocomplete search. We will implement this auto suggest text search on your textbox and also see how to set the searchable text on the textbox step by step.
Step 1: Create one table like calendar_event_master
and store some sample data which we will use for demo purpose.
CREATE TABLE `calendar_event_master` (
`event_id` int(11) NOT NULL AUTO_INCREMENT,
`event_name` varchar(255) DEFAULT NULL,
`event_start_date` date DEFAULT NULL,
`event_end_date` date DEFAULT NULL,
PRIMARY KEY (`event_id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
insert into `calendar_event_master`(`event_id`,`event_name`,`event_start_date`,`event_end_date`) values (1,'Test Event1','2022-10-01','2022-10-02');
insert into `calendar_event_master`(`event_id`,`event_name`,`event_start_date`,`event_end_date`) values (2,'Test Event2','2022-10-13','2022-10-20');
insert into `calendar_event_master`(`event_id`,`event_name`,`event_start_date`,`event_end_date`) values (3,'Test Event3','2022-10-16','2022-10-27');
Step 2: Now you need to create one simple index.php file add below code.
<!doctype html>
<html lang="en">
<head>
<title>How to make autocomplete search with PHP and AJAX</title>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" />
<meta name="viewport" content="width=device-width" />
<!-- *Note: You must have internet connection on your laptop or pc other wise below code is not working -->
<!-- bootstrap css and js -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"/>
<!-- JS for jQuery -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<!-- jQuery UI -->
<link rel="stylesheet" href="https://ajax.googleapis.com/ajax/libs/jqueryui/1.12.1/themes/smoothness/jquery-ui.css">
<script src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.12.1/jquery-ui.min.js"></script>
</head>
<body>
<br><br>
<div class="container">
<div class="row">
<div class="col-lg-6">
<div class="form-group">
<label for="event_name">Event name</label>
<input type="text" class="form-control" name="event_name" id="event_name" placeholder="Search event name">
<input type="hidden" name="event_id" id="event_id" value="">
</div>
</div>
</div>
</div>
<br>
<center>Developed by <a href="https://shinerweb.com/">Shinerweb</a></center>
<script type="text/javascript">
$( function() {
$( "#event_name" ).autocomplete({
source: function( request, response ) {
// Fetch data
$.ajax({
url: "search_data.php",
type: 'post',
dataType: "json",
data: {
search: request.term
},
success: function( data ) {
response( data );
}
});
},
select: function (event, ui) {
// Set selection
$('#event_name').val(ui.item.label); // display the selected text
$('#event_id').val(ui.item.value); // save selected id to input
return false;
},
focus: function(event, ui){
$( "#event_name" ).val( ui.item.label );
$( "#event_id" ).val( ui.item.value );
return false;
},
});
});
function split( val ) {
return val.split( /,\s*/ );
}
function extractLast( term ) {
return split( term ).pop();
}
</script>
</body>
</html>
In above code we have added some CSS and jQuery library which helpful for autocomplete search box functionality. We have added bootstrap css and js library for some designing purpose and the most important jquery and jquery-ui will use to implement autocomplete widget on your page.
Step 3: Create one connection file like database_connection.php and enter your database credentials.
<?php
$hostname = "localhost";
$username = "your_user";
$password = "your_password";
$database = "your_database";
$con=mysqli_connect($hostname,$username,$password,$database);
?>
Step 4: Finally you need to create one more php file like search_data.php to get the data from the database.
<?php
include "database_connection.php";
if(isset($_POST['search'])){
$search = mysqli_real_escape_string($con,$_POST['search']);
$query = "SELECT * FROM calendar_event_master WHERE event_name like'%".$search."%'";
$result = mysqli_query($con,$query);
$response = array();
while($row = mysqli_fetch_array($result) ){
$response[] = array("value"=>$row['event_id'],"label"=>$row['event_name']);
}
echo json_encode($response);
}
exit;
?>
In above code firstly we have include database connection and after that we have write code to get data from the database. Once data fetch from the database then we will sent as a response and that response data we will set in to the autocomplete search box.