In this blog, we will learn how to Export data into Excel in CodeIgniter. Export data functionality makes your web application user-friendly and helps the user to maintain list data. There are various file formats are available to export data and download it as a file. Microsoft Excel is a widely used spreadsheet format that organizes and maintains data.
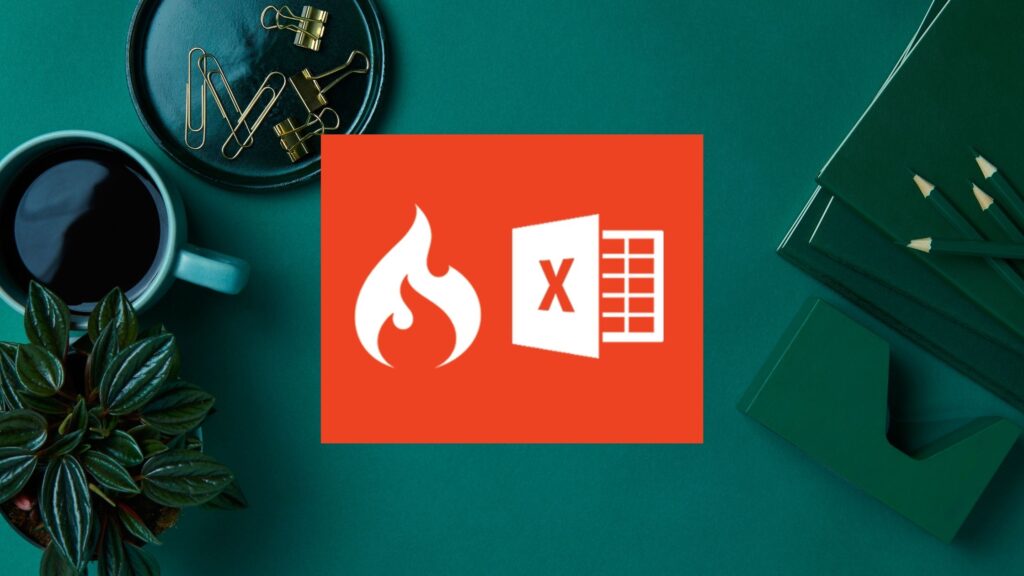
Step 1: Download PHP_XLSXWriter Library.
This library is designed to be lightweight, and have minimal memory usage.
Step 2: Copy paste above downloaded file xlsxwriter.class.php inside application/models folder
Step 3: Now create one view file like swiftcode_report_view.php inside application/views folder and write below code
<form method="post" action="https://app.shinerweb.com/index.php/bankcode/download_swift_code">
<input type="submit" value="Download SWIFT Code Report">
</form>

Step 4 : Now create one file bankcode.php inside your application/controllers folder and write below code
<?php
class bankcode extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->helper(array('form', 'url'));
$this->load->library("pagination");
$this->load->model('bankcode_model');
}
function swift_code_report()
{
$this->load->view('swiftcode_report_view');
}
function download_swift_code()
{
$this->bankcode_model->download_swift_code();
}
}
?>
You can access your view page with this link: https://app.shinerweb.com/index.php/bankcode/swift_code_report
Step 5: Once your view and controller code completed after that you need to create one more file bankcode_model.php inside your application/models folder
<?php
error_reporting(E_ALL ^ E_DEPRECATED);
class bankcode_model extends CI_Model
{
function download_swift_code()
{
$query=$this->db->query("SELECT BANK, CITY, SWIFT_CODE
FROM BANK_SWIFT_CODE"); //take your table here
$i=0;
$cnt = 2;
$rowcount = $query->num_rows();
if($rowcount != 0)
{
include_once("xlsxwriter.class.php");
ini_set('display_errors', 0);
ini_set('log_errors', 1);
error_reporting(E_ALL & ~E_NOTICE);
$filename = "SWIFT_Code_Report.xlsx";
header('Content-disposition: attachment; filename="'.XLSXWriter::sanitize_filename($filename).'"');
header("Content-Type: application/vnd.openxmlformats-officedocument.spreadsheetml.sheet");
header('Content-Transfer-Encoding: binary');
header('Cache-Control: must-revalidate');
header('Pragma: public');
$arr=array();
foreach ($query->result_array() as $row)
{
$arr[$i][0]=$row['BANK'];//your table column fields
$arr[$i][1]=$row['CITY'];
$arr[$i][2]=$row['SWIFT_CODE'];
$i=$i+1;
}
$header = array(
'Bank'=>'string',
'City'=>'string',
'SWIFT Code'=>'string'
);
//for date and time 'DD/MM/YYYY hh:mm'
//for number value ''integer''
$writer = new XLSXWriter();
$writer->setAuthor('Divyesh Patel');
$writer->writeSheetHeader('Sheet1', $header, $col_options = array('fill'=>'#699E96','font-style'=>'bold','color'=>'#ffffff', 'border'=>'left,right,top,bottom', 'border-style'=>'thin', 'height'=>15,'wrap_text'=>true,'valign'=>'center','halign'=>'center') );
$border = array( 'border'=>'left,right,top,bottom');
$border_style = array( 'border-style'=>'thin');
foreach($arr as $row)
$writer->writeSheetRow('Sheet1', $row, $border, $border_style);
$writer->writeToStdOut();
exit(0);
}
else
{
echo "<script>alert('No record(s) found.') </script>";
// Redircet to Home Page
echo "<script language=\"javascript\">window.location = '". site_url('/bankcode/swift_code_report')."' </script>";
}
}
//end function
}
?>
For more details please click here