In this blog we will create live demo for secure Firebase Login and Registration. You can use Firebase Authentication to allow users to sign in to your app using one or more sign-in methods, including email address and password.
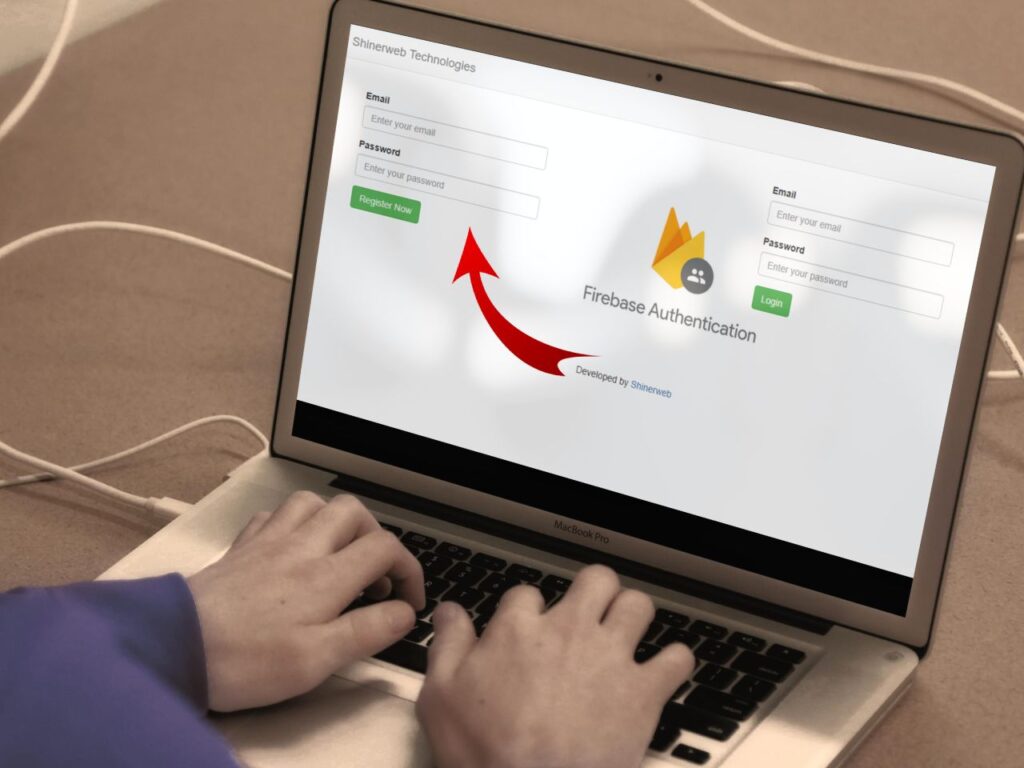
Let’s start step by step to configuration and coding for Firebase Authentication.
Step 1: Go to Firebase console and click on Add new project.
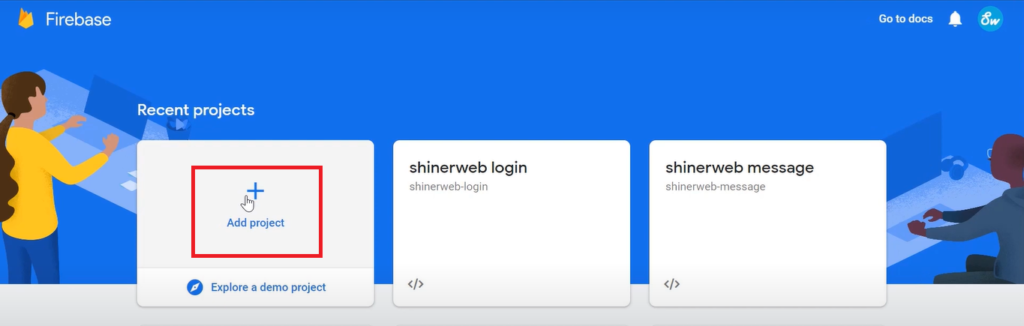
Step 2: Provide your Project name.
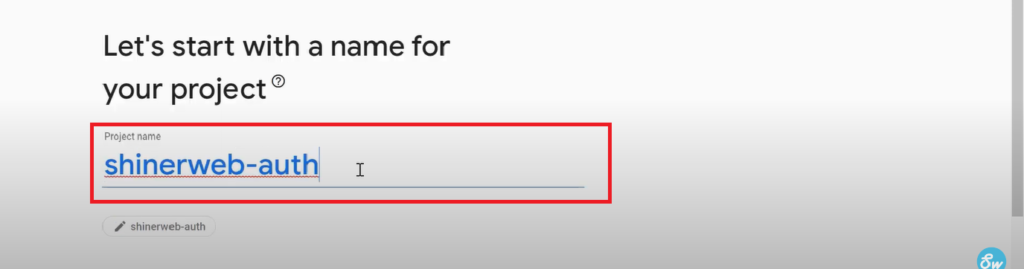
Step 3: Click on Continue to create project.
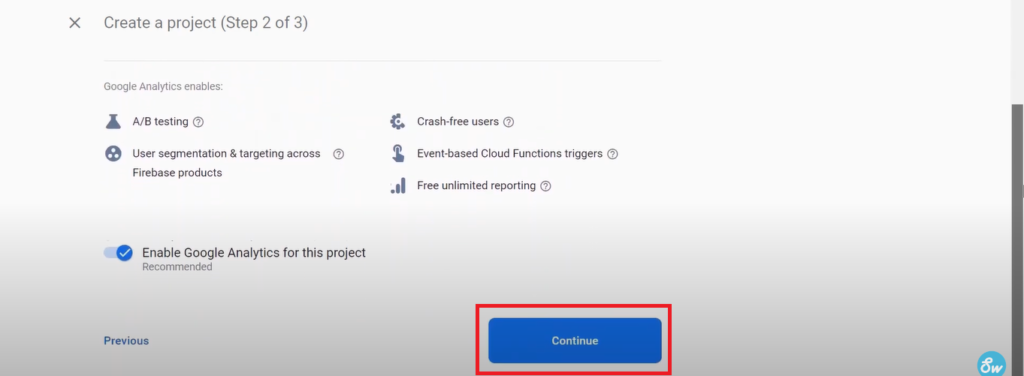
Step 4: Select your Google Analytics Account and click on Create Project.
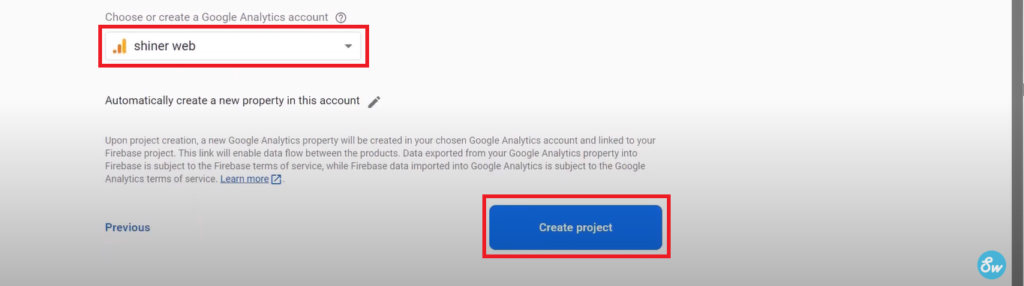
Step 5: Once your project created then you need to create Firebase App.
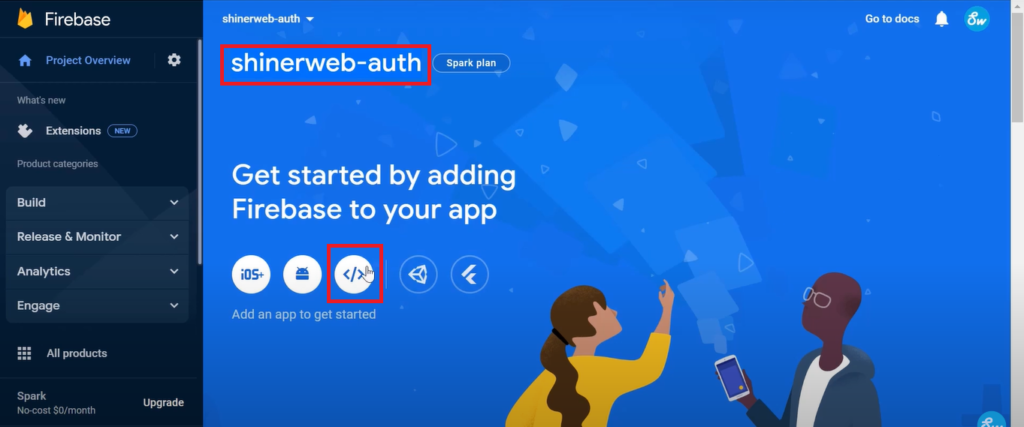
Step 6: Add Firebase to your web app.
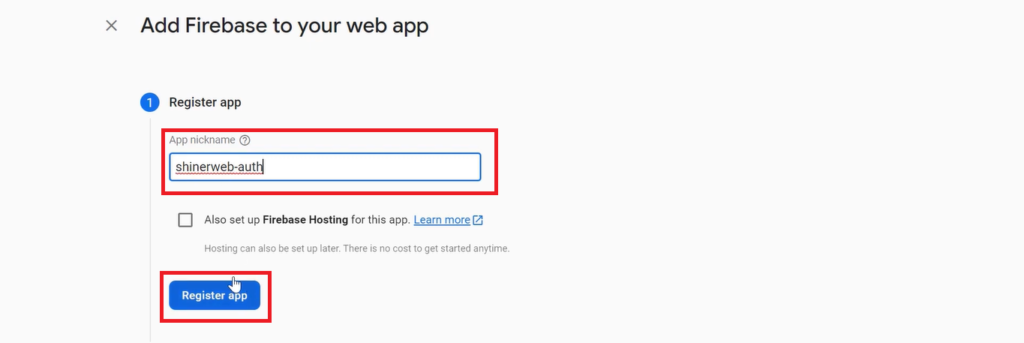
Step 7: You can save this code which will be helpful for further coding.
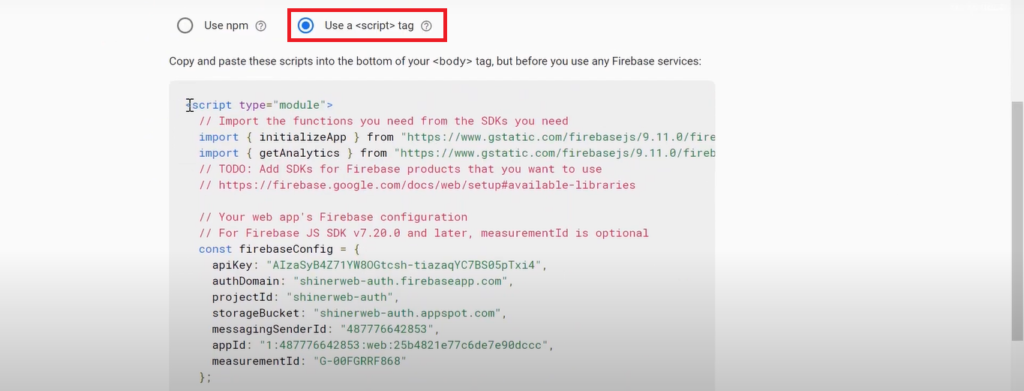
Step 8: Now you need to create one webpage like auth.html
and you can write below code.
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script>
<!-- Bootstrap theme -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<title>How to create Firebase login and register?</title>
</head>
<body>
<div class="navbar navbar-default">
<div class="navbar-header">
<a class="navbar-brand" href="https://www.shinerweb.com">Shinerweb Technologies</a>
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target=".navbar-collapse" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
</div>
<div class="navbar-collapse collapse" >
<ul class="nav navbar-nav navbar-right">
<li><a href="#" id="logout" style="display: none;">Log Out</a></li>
</ul>
</div>
</div>
<br>
<div class="container">
<form name="registration_form" id="registration_form" method="post" action="#" enctype="multipart/form-data" >
<div class="row">
<div class="col-sm-4">
<div class="form-group">
<label for="email">Email</label>
<input type="text" name="email" id="email" class="form-control" placeholder="Enter your email">
</div>
<div class="form-group">
<label for="password" >Password</label>
<input type="password" name="password" id="password" class="form-control" placeholder="Enter your password">
</div>
<button type="button" id="register" name="register" class="btn btn-success">Register Now</button>
</div><!-- end col -->
</form>
<div class="col-sm-4">
<img src="firebase_auth.png">
</div>
<form name="login_form" id="login_form" method="post" action="#" enctype="multipart/form-data" >
<div class="col-sm-4">
<div class="form-group">
<label for="email">Email</label>
<input type="text" name="login_email" id="login_email" class="form-control" placeholder="Enter your email">
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" name="login_password" id="login_password" class="form-control" placeholder="Enter your password">
</div>
<button type="button" id="login" name="login" class="btn btn-success">Login</button>
</div><!-- end col -->
</div><!-- end row -->
</form>
</div>
<br>
<center>Developed by <a href="https://shinerweb.com/">Shinerweb</a></center>
</body>
<script type="module">
// Import the functions you need from the SDKs you need
import { initializeApp } from "https://www.gstatic.com/firebasejs/9.10.0/firebase-app.js";
import { getAnalytics } from "https://www.gstatic.com/firebasejs/9.10.0/firebase-analytics.js";
import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword, signOut } from "https://www.gstatic.com/firebasejs/9.10.0/firebase-auth.js";
// TODO: Add SDKs for Firebase products that you want to use
// https://firebase.google.com/docs/web/setup#available-libraries
// Your web app's Firebase configuration
// For Firebase JS SDK v7.20.0 and later, measurementId is optional
const firebaseConfig = {
apiKey: "AIzaSyB4Z71YW8OGtcsh-tiazaqYC7BS05pTxi4",
authDomain: "shinerweb-auth.firebaseapp.com",
projectId: "shinerweb-auth",
storageBucket: "shinerweb-auth.appspot.com",
messagingSenderId: "487776642853",
appId: "1:487776642853:web:25b4821e77c6de7e90dccc",
measurementId: "G-00FGRRF868"
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
const analytics = getAnalytics(app);
const auth = getAuth();
console.log(app);
//----- New Registration code start
document.getElementById("register").addEventListener("click", function() {
var email = document.getElementById("email").value;
var password = document.getElementById("password").value;
//For new registration
createUserWithEmailAndPassword(auth, email, password)
.then((userCredential) => {
// Signed in
const user = userCredential.user;
console.log(user);
alert("Registration successfully!!");
// ...
})
.catch((error) => {
const errorCode = error.code;
const errorMessage = error.message;
// ..
console.log(errorMessage);
alert(error);
});
});
//----- End
//----- Login code start
document.getElementById("login").addEventListener("click", function() {
var email = document.getElementById("login_email").value;
var password = document.getElementById("login_password").value;
signInWithEmailAndPassword(auth, email, password)
.then((userCredential) => {
// Signed in
const user = userCredential.user;
console.log(user);
alert(user.email+" Login successfully!!!");
document.getElementById('logout').style.display = 'block';
// ...
})
.catch((error) => {
const errorCode = error.code;
const errorMessage = error.message;
console.log(errorMessage);
alert(errorMessage);
});
});
//----- End
//----- Logout code start
document.getElementById("logout").addEventListener("click", function() {
signOut(auth).then(() => {
// Sign-out successful.
console.log('Sign-out successful.');
alert('Sign-out successful.');
document.getElementById('logout').style.display = 'none';
}).catch((error) => {
// An error happened.
console.log('An error happened.');
});
});
//----- End
</script>
</html>
In above code we have design one registration and login form by using some simple html code.
The most important you need to import some firebase library which is required for Firebase authentication. Don’t forgot to include below library inside script tag.
import { initializeApp } from "https://www.gstatic.com/firebasejs/9.10.0/firebase-app.js";
import { getAnalytics } from "https://www.gstatic.com/firebasejs/9.10.0/firebase-analytics.js";
import { getAuth, createUserWithEmailAndPassword, signInWithEmailAndPassword, signOut } from "https://www.gstatic.com/firebasejs/9.10.0/firebase-auth.js";
We are using createUserWithEmailAndPassword
method to register a new user. For login signInWithEmailAndPassword
method use.
Step 9: Finally you need to enable Sing-in method.
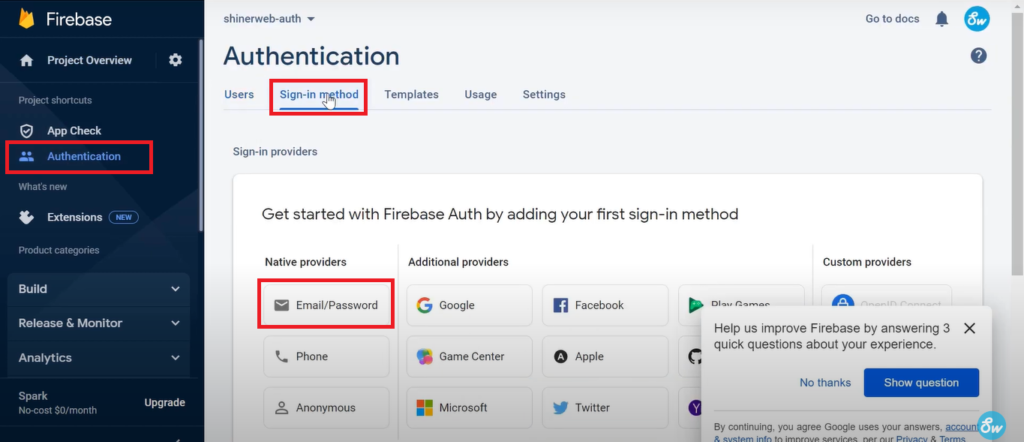
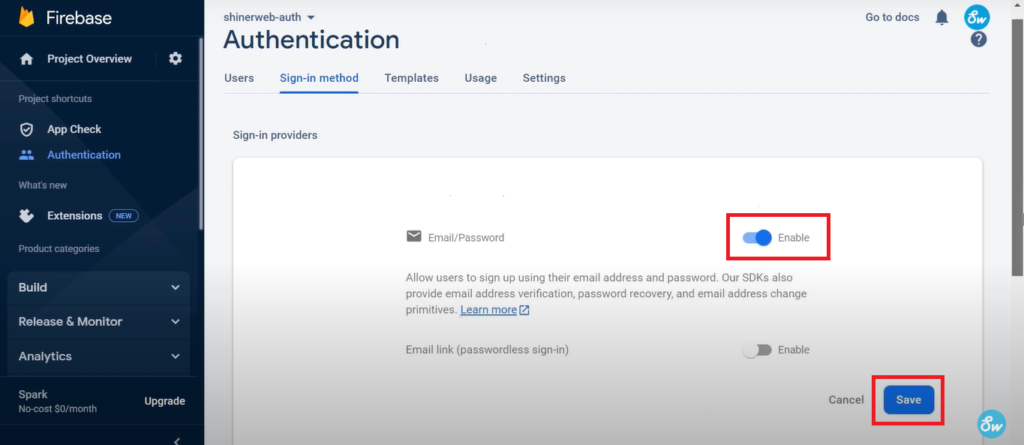