In this blog we will create live example for sweet alert. Sweet Alert is a method to customize alerts in your applications, websites and games. A beautiful, responsive, customizable and accessible replacement for JavaScript’s popup boxes.
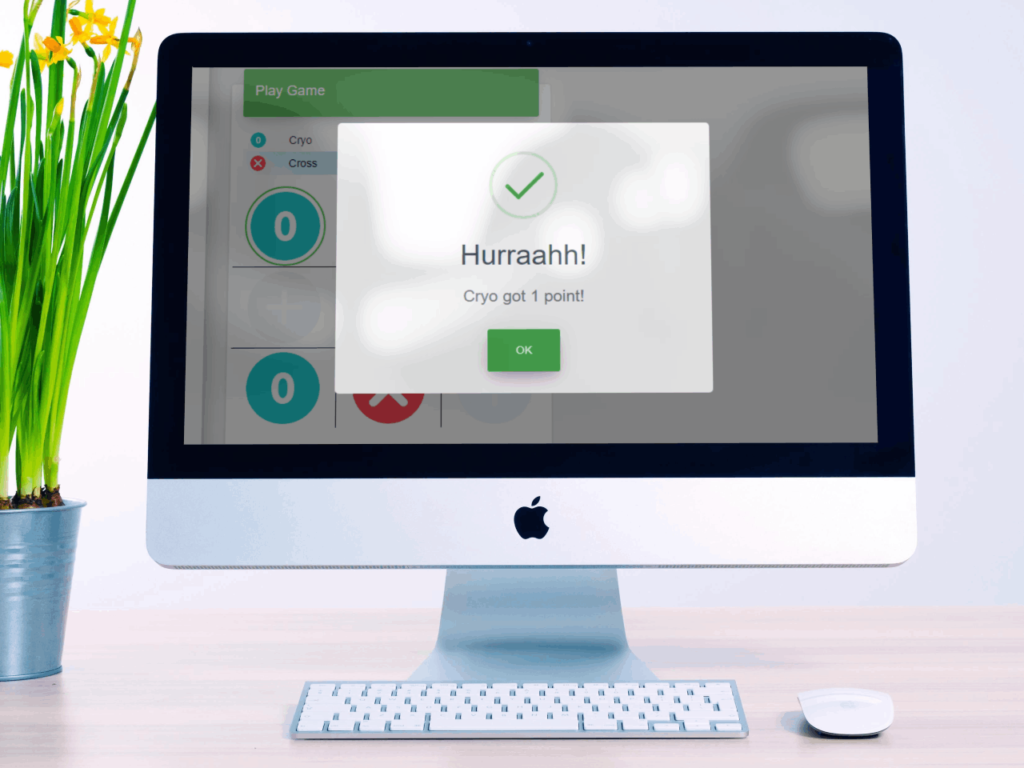
What is a sweet alert?
Sweet Alert is a way to customize alerts in your games and websites. It allows you to change from a standard JavaScript button. We can add buttons, change the color text, and even add additional alerts that change depending on user click.
Let’s start step by step configuration and create live example to understand how sweet alert work.
Step 1: Create one HTML file and include below library.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script>
<script src="https://unpkg.com/sweetalert/dist/sweetalert.min.js" ></script>
Step 2: Create one simple button and call one function with onclick method like onclick="function1()"
<button type="button" class="btn btn-primary" onclick="function1()">Basic message</button>
Step 3: Finally you need to call your sweet alert message with below code.
<script language="JavaScript">
function function1() {
swal("Hello world!");
}
</script>
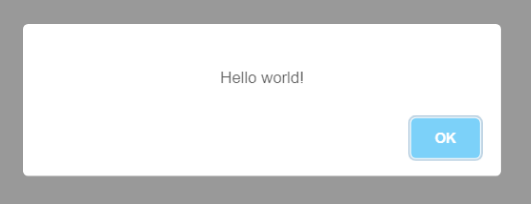
So this is how you can simply configure sweet alert. Now let’s see some another examples of sweetAlert.
Create sweet alert with title and text
<button type="button" class="btn btn-secondary" onclick="function2()">Title with a text</button>
<script language="JavaScript">
function function2() {
swal("Success!", "Your data have been saved. Thank you!");
}
</script>
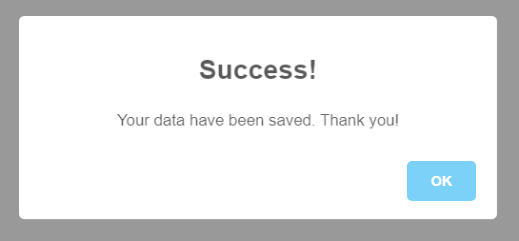
In above example you need to pass two arguments and one is for title and second as a text.
Create success and error icon in sweet alert with title and text
<button type="button" class="btn btn-success" onclick="function3()">Success with icon</button>
<button type="button" class="btn btn-danger" onclick="function4()">Error with icon</button>
<script language="JavaScript">
function function3() {
swal("Success!", "Your data have been saved. Thank you!", "success");
}
function function4() {
swal("Sorry!", "Opps, something went wrong. Please try again later.", "error");
}
</script>
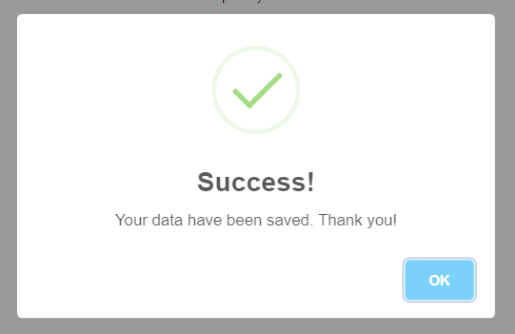
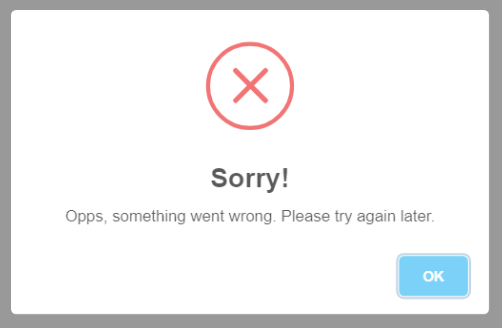
In above example you must have to pass third parameter as "success"
and "error"
to display animated icons.
Display confirm message before you delete or save something
<button type="button" class="btn btn-warning" onclick="function5()">With confirm button</button>
function function5() {
swal({
title: "Are you sure?",
text: "Once deleted, you will not be able to recover this file!",
icon: "warning",
buttons: true,
dangerMode: true,
})
.then((willDelete) => {
if (willDelete) {
swal("Poof! Your file has been deleted!", {
icon: "success",
});
} else {
swal("Your file is safe!");
}
});
}
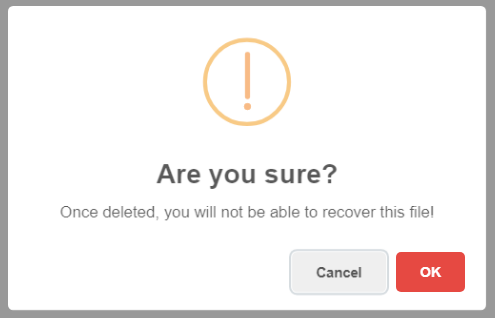
This comes in handy if you want to warn the user before they perform a dangerous action.
A message with a custom icon or image
<button type="button" class="btn btn-info" onclick="function6()">With image</button>
function function6() {
swal({
title: "Sweet!",
text: "Here's a custom image.",
icon: "https://picsum.photos/id/1/200/300"
});
}
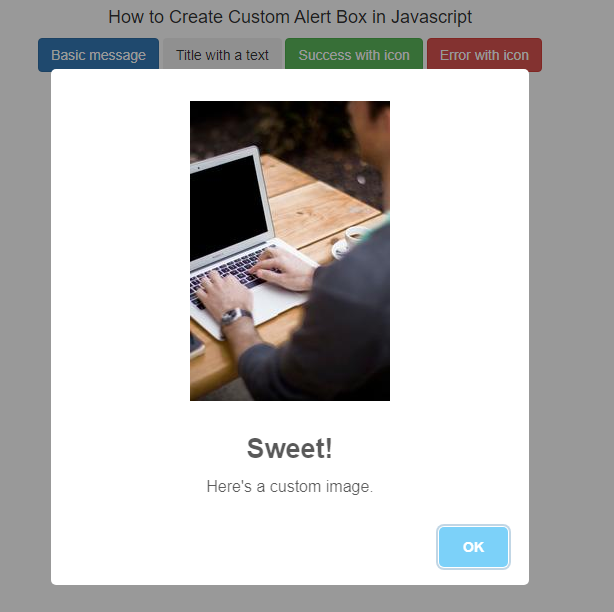
In above example we display one image with some message text.