Here we validate end date should be greater than start date using jQuery. Most of time when we use date range, we always need to validate two dates.
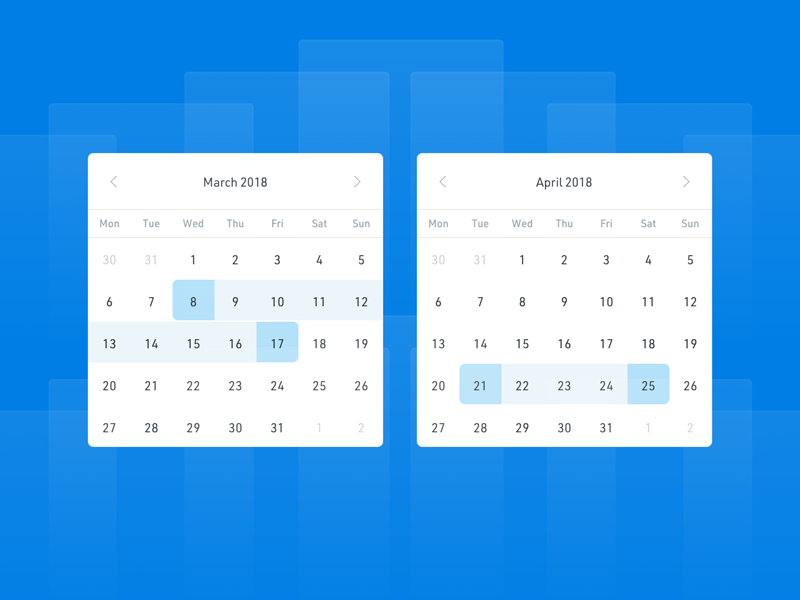
Step 1. Make a HTML file and add one textbox with id=”from_date” and id=”to_date”
<!DOCTYPE html>
<html>
<head>
<title>how to create from and to date range date picker</title>
</head>
<body>
From date <input type="text" name="from_date" id="from_date" placeholder="From date">
To date <input type="text" name="to_date" id="to_date" placeholder="To date">
</body>
</html>
Step 2: After that you need to add below css and js files inside <head> tag.
<link rel="stylesheet" href="//code.jquery.com/ui/1.8.24/themes/base/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.8.2.js"></script>
<script src="//code.jquery.com/ui/1.8.24/jquery-ui.js"></script>
Step 3: Finally add below code to call datepicker
<script type="text/javascript">
$(document).ready(function(){
$( "#from_date" ).datepicker({
minDate: 0,
dateFormat: 'yy-mm-dd',
changeMonth: true,
changeYear: true,
onClose: function(selectedDate) {
// Set the minDate of 'to' as the selectedDate of 'from'
$("#to_date").datepicker("option", "minDate", selectedDate);
}
});
$( "#to_date" ).datepicker({
minDate: 0,
dateFormat: 'yy-mm-dd',
changeMonth: true,
changeYear: true
});
});
</script>
Finally your from and to date picker is ready. If you want to see live output then click on below link.
Full code as below:
<!DOCTYPE html>
<html>
<head>
<title>how to create from and to date range date picker</title>
<!-- *Note: You must have internet connection on your laptop or pc otherwise below code is not working -->
<link rel="stylesheet" href="//code.jquery.com/ui/1.8.24/themes/base/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.8.2.js"></script>
<script src="//code.jquery.com/ui/1.8.24/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$( "#from_date" ).datepicker({
minDate: 0,
dateFormat: 'yy-mm-dd',
changeMonth: true,
changeYear: true,
onClose: function(selectedDate) {
// Set the minDate of 'to' as the selectedDate of 'from'
$("#to_date").datepicker("option", "minDate", selectedDate);
}
});
$( "#to_date" ).datepicker({
minDate: 0,
dateFormat: 'yy-mm-dd',
changeMonth: true,
changeYear: true
});
});
</script>
</head>
<body>
<h4>how to create from and to date range date picker <a href="https://www.shinerweb.com">Demo by Shinerweb.com</a></h4>
From date <input type="text" name="from_date" id="from_date" placeholder="From date">
To date <input type="text" name="to_date" id="to_date" placeholder="To date">
</body>
</html>