The datepicker is tied to a standard form input field. Focus on the input (click, or use the tab key) to open an interactive calendar in a small overlay.
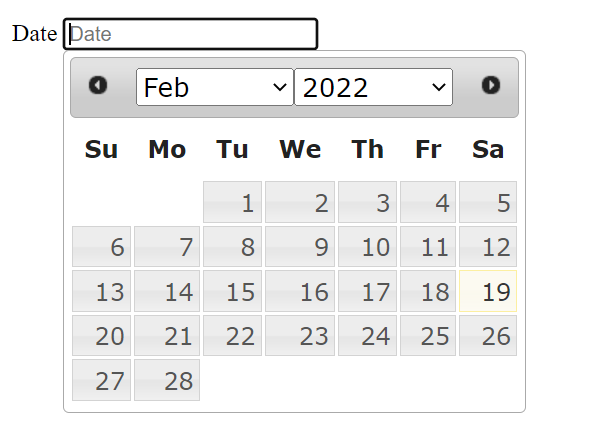
Step 1. Make a HTML file and add one textbox with id=”datepicker”
<!DOCTYPE html>
<html>
<head>
<title>How to add datepicker in jquery</title>
</head>
<body>
<h1>How to add datepicker in jquery</h1>
Date <input type="text" name="datepicker" id="datepicker" placeholder="Date">
</body>
</html>
Step 2: After that you need to add below css and js files inside <head> tag.
<link rel="stylesheet" href="//code.jquery.com/ui/1.8.24/themes/base/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.8.2.js"></script>
<script src="//code.jquery.com/ui/1.8.24/jquery-ui.js"></script>
Step 3: Finally add below code to call datepicker
<script type="text/javascript">
$("#datepicker").live('click',function() {
$(this).datepicker({
dateFormat: "yy-mm-dd",
changeMonth: true,
changeYear: true
}).datepicker( "show" );
});
</script>
Finally your datepicker is ready. If you want to see live output then click on below link.
Here is full code available.
<!DOCTYPE html>
<html>
<head>
<title>How to add datepicker in jquery</title>
<!-- *Note: You must have internet connection on your laptop or pc otherwise below code is not working -->
<link rel="stylesheet" href="//code.jquery.com/ui/1.8.24/themes/base/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.8.2.js"></script>
<script src="//code.jquery.com/ui/1.8.24/jquery-ui.js"></script>
<script type="text/javascript">
$("#datepicker").live('click',function() {
$(this).datepicker({
dateFormat: "yy-mm-dd",
changeMonth: true,
changeYear: true
}).datepicker( "show" );
});
</script>
</head>
<body>
<h1>How to add datepicker in jquery <a href="https://www.shinerweb.com">Demo by Shinerweb.com</a></h1>
Date <input type="text" name="datepicker" id="datepicker" placeholder="Date">
</body>
</html>